XML Interface
Introduction
When working with XML documents, Creo Elements/Direct Modeling maintains
an representation of the documents on the C++ level. The functions below
provide an interface to read and manipulate these documents.
Note that the objects are not automatically garbage-collected. It
is recommended to call the function sd-xml-clear-cache after processing of the XML
document is done.
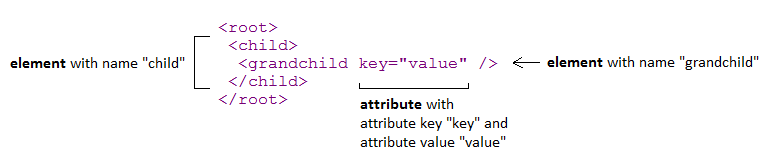
Example
Detailed example Lisp code about reading, writing and processing XML can
be found here.
Functions
Read / Write XML files
Processing XML documents
Processing XML elements
Miscelleanous

SD-XML-READ-FILE [function]
(sd-xml-read-file filename)
- Description:
- Reads an XML document from a file.
-
- Parameters:
- filename {STRING} - the name of the file to read
-
- Return Value:
- document {XML DOCUMENT} - reference to the XML document
- nil - failure
-
- Example:
-
(setf my-xml-document (sd-xml-read-file "d:/temp/document.xml"))

SD-XML-WRITE-FILE [function]
(sd-xml-write-file document filename :pretty-print pretty-print :encoding encoding)
- Description:
- Writes an XML document to a file.
-
- Parameters:
-
- document {XML DOCUMENT} - reference to the XML
document
- filename {STRING} - name of the file to write
- :pretty-print {BOOLEAN [t]} - optional parameter to write
formatted output with line breaks and indentation
- :encoding {STRING ["UTF-8"]} - optional parameter to
specify the encoding
- legal values are "UTF-8" and "UTF-16"
- Return Value:
- t - success
- nil - failure
-
- Example:
-
(setf result (sd-xml-write-file my-xml-document "d:/temp/document.xml"))

SD-XML-GET-ROOT-NAME [function]
(sd-xml-get-root-name filename)
- Description:
- Reads only the root name of an XML document in a file. Reading only
the root name is much more efficient than reading whole document and
inquiring then the name of the root element. This function might be very
useful if specific handlers are registered for xml files with certain root
name.
-
- Parameters:
- filename {STRING} - the name of the file to read
-
- Return Value:
- name {STRING} - the root name
-
- Example:
-
(setf root-name (sd-xml-get-root-name "d:/temp/document.xml"))
=> "root"

SD-XML-SERIALIZE [function]
(sd-xml-serialize document)
- Description:
- Serializes an XML document into a string.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
-
- Return Value:
- content {STRING} - the document content as a string
-
- Example:
-
(setf content (sd-xml-serialize my-xml-document))
=> "<root><element1/></root>"

SD-XML-DESERIALIZE [function]
(sd-xml-deserialize content)
- Description:
- Creates an XML document from a string.
-
- Parameters:
- content {STRING} - the document content as a string
-
- Return Value:
- document {XML DOCUMENT} - an XML document
-
- Example:
-
(setf my-xml-document (sd-xml-deserialize "<root><element1/></root>"))

SD-XML-CREATE-DOCUMENT [function]
(sd-xml-create-document)
- Description:
- Creates a new document object in memory.
-
- Return Value:
- document {XML DOCUMENT} - pointer to the XML document
-
- Example:
-
(setf my-xml-document (sd-xml-create-document))

SD-XML-DOCUMENT-GET-ROOT [function]
(sd-xml-document-get-root document)
- Description:
- Gets the root element of the document.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
-
- Return Value:
- element {XML ELEMENT} - the root XML element
-
- Example:
-
(setf root-element (sd-xml-document-get-root my-xml-document))

SD-XML-DOCUMENT-SET-ROOT [function]
(sd-xml-document-set-root document element)
- Description:
- Sets the root element of the document.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
- element {XML ELEMENT} - the new root XML element
-
- Return Value:
- element {XML ELEMENT} - the root element
-
- Example:
-
(setf root-element (sd-xml-document-set-root my-xml-document element))

SD-XML-DOCUMENT-SELECT-ELEMENT [function]
(sd-xml-document-select-element document xpath)
- Description:
- Gets a document element by its XPath.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
- xpath {STRING} - the XPath of the element, might be single
element path or element which has a certain attribute of specific
value
-
- Return Value:
- element {XML ELEMENT} - the selected element
-
- Example:
-
(setf element (sd-xml-document-select-element my-xml-document "/root/element1"))
(setf element (sd-xml-document-select-element my-xml-document "/root/element1[@key1='value1']"))

SD-XML-CREATE-ELEMENT [function]
(sd-xml-create-element document name)
- Description:
- Creates a new XML element with specified name.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
- name {STRING} - name of the element
-
- Return Value:
- element {XML ELEMENT} - the created XML element
-
- Example:
-
(setf my-xml-element (sd-xml-create-element my-xml-document "element1"))

SD-XML-ELEMENT-GET-NAME [function]
(sd-xml-element-get-name element)
- Description:
- Gets the name of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire the name
from
-
- Return Value:
- name {STRING} - the element name
-
- Example:
-
(setf element-name (sd-xml-element-get-name my-xml-element))
=> "element1"

SD-XML-ELEMENT-GET-CHILDREN [function]
(sd-xml-element-get-children element)
- Description:
- Gets all children of the element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
-
- Return Value:
- children {List of XML ELEMENTs} - a list of child elements
-
- Example:
-
(setf element-children (sd-xml-element-get-children my-xml-element))

SD-XML-ELEMENT-GET-NAMED-CHILDREN [function]
(sd-xml-element-get-named-children element name)
- Description:
- Gets all children of the element with a given name.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
- name {STRING} - the name of the children to inquire
-
- Return Value:
- children {List of XML ELEMENTs} - a list of child elements with
specified name
-
- Example:
-
(setf element-children (sd-xml-element-get-named-children my-xml-element "element2"))

SD-XML-ELEMENT-GET-NAMED-CHILD [function]
(sd-xml-element-get-named-child element name)
- Description:
- Gets the first child of the element with a given name. It is
basically the same function like sd-xml-element-get-named-children.
Only the first child element is returned.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
- name {STRING} - the name of the child to inquire
-
- Return Value:
- element {XML ELEMENT} - the first child element with this
name
-
- Example:
-
(setf element-child (sd-xml-element-get-named-child my-xml-element "element2"))

SD-XML-ELEMENT-ADD-CHILD [function]
(sd-xml-element-add-child parent-element child-element)
- Description:
- Adds a child XML element to a given parent XML element.
-
- Parameters:
- parent-element {XML ELEMENT}
- child-element {XML ELEMENT}
-
- Return Value:
- t - success
- nil - failure
-
- Example:
-
(setf result (sd-xml-element-add-child my-xml-element child-element))

SD-XML-ELEMENT-GET-TEXT [function]
(sd-xml-element-get-text element)
- Description:
- Gets the text content of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
-
- Return Value:
- content {STRING} - the text content
-
- Example:
-
(setf text (sd-xml-element-get-text my-xml-element))
=> "XML element value"

SD-XML-ELEMENT-SET-TEXT [function]
(sd-xml-element-set-text element text)
- Description:
- Sets the text content of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to change the content
- text {STRING} - the new text content
-
- Return Value:
- t - success
- nil - failure
-
- Example:
-
(setf result (sd-xml-element-set-text my-xml-element "XML element value"))

SD-XML-ELEMENT-GET-ATTRIBUTES [function]
(sd-xml-element-get-attributes element)
- Description:
- Gets the keys of all attributes of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
-
- Return Value:
- attributes {List of STRINGs} - a list of attribute keys
- nil - element does not have any attribute
-
- Example:
-
(setf attribute-keys (sd-xml-element-get-attributes my-xml-element))
=> ("key1" "key2")

SD-XML-ELEMENT-GET-ATTRIBUTE [function]
(sd-xml-element-get-attribute element attribute-key)
- Description:
- Gets the attribute value of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
- attribute-key {STRING} - the attribute key to inquire
-
- Return Value:
- value {STRING} - the attribute value
- nil - the attribute is not set
-
- Example:
-
(setf attribute-value (sd-xml-element-get-attribute my-xml-element "key1"))
=> "value1"

SD-XML-ELEMENT-SET-ATTRIBUTE [function]
(sd-xml-element-set-attribute element attribute-key attribute-value)
- Description:
- Sets the attribute value of an XML element for a specified attribute
key.
-
- Parameters:
- element {XML ELEMENT} - the element to change
- attribute-key {STRING} - the attribute key to change the
value
- attribute-value {STRING} - the new attribute value
-
- Return Value:
- t - success
- nil - failure
-
- Example:
-
(setf result (sd-xml-element-set-attribute my-xml-element "key1" "value_modified"))

SD-XML-ELEMENT-GET-PARENT [function]
(sd-xml-element-get-parent element)
- Description:
- Gets the parent of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
-
- Return Value:
- element {XML ELEMENT} - the parent element
-
- Example:
-
(setf element-parent (sd-xml-element-get-parent my-xml-element))

SD-XML-ELEMENT-GET-DOCUMENT [function]
(sd-xml-element-get-document element)
- Description:
- Gets the owner document of an XML element.
-
- Parameters:
- element {XML ELEMENT} - the element to inquire
-
- Return Value:
- document {XML DOCUMENT} - the owner document
-
- Example:
-
(setf my-xml-document (sd-xml-element-get-document my-xml-element))

SD-XML-TRANSFORM-FILE [function]
(sd-xml-transform-file document stylesheet)
- Description:
- Applies an XSLT transformation
to an XML document.
-
- Parameters:
- document {XML DOCUMENT} - reference to the XML document
- stylesheet {STRING} - path to a stylesheet file
-
- Return Value:
- Document {XML DOCUMENT} - the transformed document
-
- Example:
-
(setf transformed-document (sd-xml-transform-file my-xml-document "d:/temp/stylesheet.xsl"))

SD-XML-CLEAR-CACHE [function]
(sd-xml-clear-cache)
- Description:
- Disposes all XML documents and elements created through the LISP
interface. LISP references to any of the objects obtained by the LISP API
will become invalid.
-
- Example:
-
(sd-xml-clear-cache)
© 2023 Parametric
Technology GmbH
(a subsidiary of PTC Inc.), All Rights Reserved |